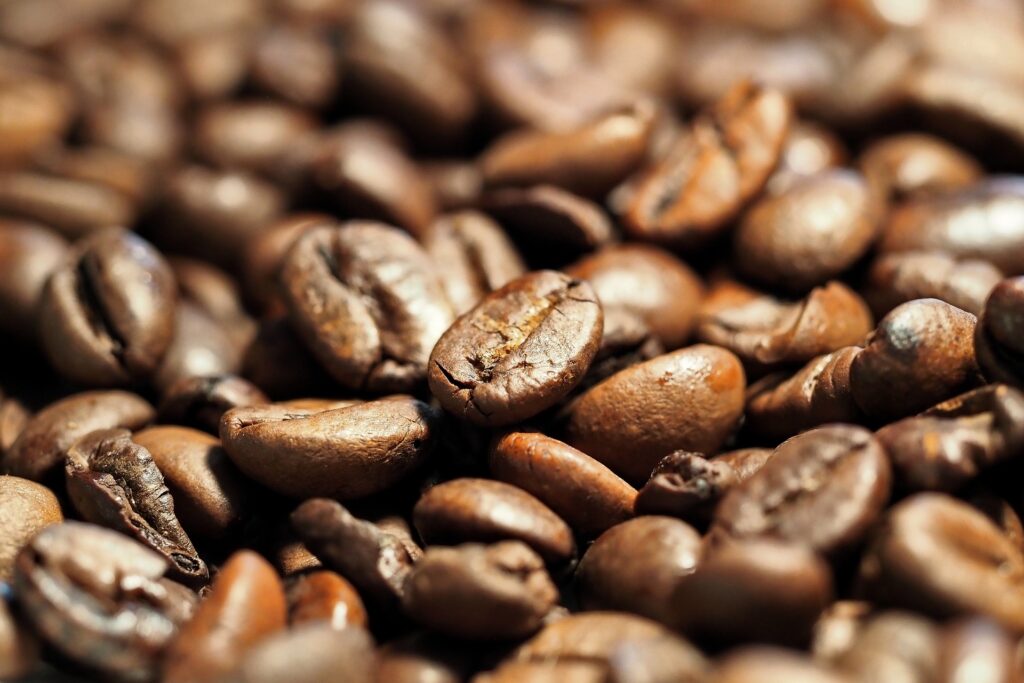
SOLID is an acronym for five essential principles in object-oriented programming and design that promote clean, maintainable, and scalable software. In this post, we’ll explore each SOLID principle, provide code examples illustrating violations and corrections, and discuss the real-world problems that can emerge when these principles are ignored.
Single Responsibility Principle (SRP):
The SRP emphasizes that a class should have only one reason to change. This principle promotes code that is easier to understand and modify because each class has a single, well-defined responsibility.
Violation Example:
class Customer { void calculateDiscount() { // Calculate discount logic } void sendEmailConfirmation() { // Send email logic } }
Explanation of Violation: The Customer
class violates SRP by handling two distinct responsibilities: calculating discounts and sending email confirmations. This creates coupling between unrelated functions and makes the class prone to change for reasons beyond its intended scope.
Correction Example:
class Customer { void calculateDiscount() { // Calculate discount logic } } class EmailService { void sendEmailConfirmation() { // Send email logic } }
Explanation of Correction: To adhere to SRP, we split the responsibilities into separate classes: Customer
for discount calculations and EmailService
for email confirmations. This separation isolates changes to specific areas of the codebase, improving maintainability and reducing the risk of unintended side effects.
Real-World Problem: SRP violations can result in maintenance nightmares. For example, if a change is made to the email logic (e.g., for compliance with new regulations), it may inadvertently impact the discount calculation, potentially causing financial discrepancies and customer dissatisfaction.
Open/Closed Principle (OCP)
The OCP emphasizes that software entities (such as classes and modules) should be open for extension but closed for modification. This principle encourages code that can be extended with new features without altering existing, tested code.
Violation Example:
class Square { int side; int calculateArea() { return side * side; } }
Explanation of Violation: The Square
class violates OCP because adding new shapes would require modifying its code. This makes the class closed for extension, as any change to accommodate new shapes would involve altering existing code.
Correction Example:
interface Shape { int calculateArea(); } class Square implements Shape { int side; @Override public int calculateArea() { return side * side; } }
Explanation of Correction: To conform to OCP, we introduce an interface, Shape
, that defines a common method for calculating area. Now, new shapes can be added by creating classes that implement the Shape
interface without altering existing code.
Real-World Problem: OCP violations can lead to tightly coupled code that’s difficult to maintain and extend. In practice, modifying existing code to add new features can introduce unintended side effects, increasing the risk of introducing bugs.
Liskov Substitution Principle (LSP)
The LSP emphasizes that objects of derived classes should be substitutable for objects of their base classes without affecting program correctness. This principle ensures that derived classes maintain the expected behavior of their base classes.
Violation Example:
class Bird { void fly() { // Fly logic } } class Ostrich extends Bird { void fly() { // Ostriches cannot fly! } }
Explanation of Violation: The Ostrich
class violates LSP by overriding the fly()
method when it cannot fly. This breaks the expected behavior of the base class, Bird
, and can lead to unexpected behavior in code that relies on the ability to fly.
Correction Example:
interface Bird { void move(); } class Sparrow implements Bird { void move() { // Fly logic for sparrows } } class Ostrich implements Bird { void move() { // Running logic for ostriches } }
Explanation of Correction: To adhere to LSP, we introduce the Bird
interface with a generic move()
method. Both Sparrow
and Ostrich
implement the interface, providing their respective move behaviors without violating the base class’s expected behavior.
Real-World Problem: LSP violations can lead to code that behaves unexpectedly when substituting derived classes for their base classes. In practice, this can result in runtime errors and software failures when code relies on consistent behavior.
Interface Segregation Principle (ISP)
The ISP emphasizes that clients should not be forced to depend on interfaces they do not use. This principle promotes the creation of small, client-specific interfaces rather than large, monolithic ones.
Violation Example:
interface Worker { void work(); void eat(); } class Robot implements Worker { void work() { // Work logic for robots } void eat() { // Robots do not eat } }
Explanation of Violation: The Robot
class violates ISP by implementing the eat()
method even though it doesn’t need it. This forces the class to depend on an unnecessary method, creating code bloat and confusion.
Correction Example:
interface Workable { void work(); } interface Eatable { void eat(); } class Robot implements Workable { void work() { // Work logic for robots } }
Explanation of Correction: To adhere to ISP, we create smaller, client-specific interfaces (Workable
and Eatable
) that better reflect the needs of the implementing classes. The Robot
class now implements only the Workable
interface, avoiding unnecessary dependencies.
Real-World Problem: ISP violations can lead to codebases filled with classes implementing methods they don’t need, resulting in increased complexity and reduced code clarity. This can make the codebase harder to understand and maintain.
Dependency Inversion Principle (DIP)
The DIP emphasizes that high-level modules should not depend on low-level modules; both should depend on abstractions. This principle encourages decoupling and promotes flexibility in software design.
Violation Example:
class LightBulb { void turnOn() { // Turn on the light bulb } } class Switch { private LightBulb bulb; Switch() { bulb = new LightBulb(); } void operate() { bulb.turnOn(); } }
Explanation of Violation: The Switch
class violates DIP by directly creating a LightBulb
instance, creating a tight coupling between high-level (Switch
) and low-level (LightBulb
) modules. This makes the code less flexible and harder to maintain.
Correction Example:
interface Switchable { void turnOn(); } class LightBulb implements Switchable { void turnOn() { // Turn on the light bulb } } class Switch { private Switchable device; Switch(Switchable device) { this.device = device; } void operate() { device.turnOn(); } }
Explanation of Correction: To conform to DIP, we introduce the Switchable
interface, allowing high-level modules to depend on abstractions. The Switch
class now accepts a Switchable
device, promoting flexibility and adhering to the principle.
Real-World Problem: DIP violations can hinder code flexibility, making it challenging to adapt to changing requirements or replace low-level components. This can lead to costly and time-consuming maintenance and upgrades.
Conclusion
Mastering the SOLID principles is essential for creating software that is not only robust but also adaptable to evolving requirements. These principles guide the design of maintainable code by promoting single responsibilities, open for extension but closed for modification, consistent behavior, minimal interfaces, and abstraction-based dependencies. Understanding and applying these principles judiciously will equip you to craft software systems that stand the test of time and maintain your authority as a proficient software engineer.