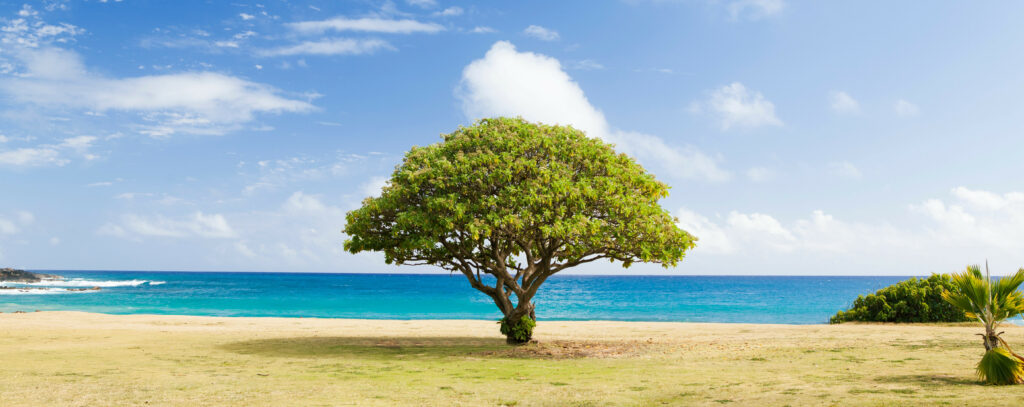
Introduction
Git is an essential tool for software development, enabling version control, effective collaboration, and detailed tracking of changes in projects. In this guide, we will explore the key aspects of Git, from cloning and initializing repositories to creating branches, using the stage, making commits, merging, pushing changes to remote repositories, and creating pull requests. We will use coherent examples throughout the guide to illustrate each concept.
Cloning or Initializing a Git Repository
To clone a remote repository:
git clone https://github.com/your-username/MyProject.git
To initialize a new local repository:
mkdir MyProject cd MyProject git init
Creating a Branch
Suppose you want to create a new branch called “feature-new” to work on a new feature. Creating branches is essential for:
- Parallel Development: Allows you to work on features or bug fixes without interfering with the main code.
- Isolation: Enables testing new features without impacting the main project.
git checkout -b feature-new
The Concept of Staging
The stage is where you prepare changes for the next commit. Let’s create a new file called “new-file.txt” and show the result of the git status
command before and after adding the file to the stage.
Before Adding to the Stage:
When creating the “new-file.txt” and checking the status before adding it to the stage, Git will indicate that the file is pending to be added. The status will show that there is an untracked file in the working directory.
# Create the new file touch new-file.txt # Check the status before adding to the stage git status
Result before adding to the stage:
On branch feature-new Untracked files: (use "git add <file>..." to include in what will be committed) new-file.txt nothing added to commit but untracked files present (use "git add" to track)
After Adding to the Stage:
Now, after adding the file to the stage using git add new-file.txt
, the status will show that the file has been added to the stage and is ready to be committed.
# Add the file to the stage git add new-file.txt # Check the status after adding to the stage git status
Result after adding to the stage:
On branch feature-new Changes to be committed: (use "git restore --staged <file>..." to unstage) new file: new-file.txt
Now, the “new-file.txt” is in the stage and ready to be included in the next commit. This allows you to select which changes you want to include in your Git version history.
Removing a File from the Stage
In addition to adding files to the stage, it is possible to remove files that were added accidentally or that you do not want to include in the next commit. To do this, use the git reset HEAD file-name
command to unstage it while keeping the local changes. If you also want to remove the file from the local repository (but keep it locally), you can use git rm --cached file-name
.
Removing the File from the Stage:
# Remove the file from the stage git reset HEAD file-name
Removing the File from the Stage and the Local Repository (Keeping the File Locally):
# Remove the file from the stage and the local repository (keeping it locally) git rm --cached file-name
Committing Changes
Once you have staged your changes, it’s time to make a commit to record them in your local repository.
# Commit the staged changes with a descriptive message git commit -m "Add new-file.txt to the project"
Pushing Changes to the Remote Repository
To share your committed changes with the remote repository, you can use the git push
command.
# Push your local changes to the remote repository git push origin feature-new
This command pushes the changes from the local branch “feature-new” to the remote repository, ensuring that your collaborators can access and review your work.
Merging Changes
In Git, the git merge
command is used to combine changes from one branch into another. Here’s how you can do it while ensuring both the main branch and the feature branch are up-to-date.
Example Scenario:
You’re working on a feature branch called “feature-login,” and you want to update it with the latest changes from the main branch before completing your feature.
Step 1: Ensure Both Branches Are Up-to-Date
Before performing the merge, make sure both the main branch and the feature branch are up-to-date with the latest changes from the remote repository.
# Switch to the main branch and update it git checkout main git pull origin main # Switch to the feature branch and update it git checkout feature-login git pull origin feature-login
Now, both branches are up-to-date with the latest changes from the remote repository.
Step 2: Perform the Merge
With both branches up-to-date, you can use the git merge
command to manually merge the changes from the main branch into your feature branch
# Merge the latest changes from the main branch into feature-login git merge main
If there are no conflicts, Git will perform an automatic merge. However, if conflicts occur (i.e., changes in the same lines of code in both branches), Git will indicate where the conflicts occur, and you’ll need to resolve them manually.
Step 3: Resolve Conflicts (if necessary):
If conflicts arise during the merge, Git will mark the conflicted areas in the affected files. You’ll need to open these files, manually resolve the conflicts, and then add the resolved files to the staging area.
# After resolving conflicts, add the resolved files git add conflicted-file1.txt conflicted-file2.txt
Step 4: Commit the Merge
Once you’ve resolved the conflicts and staged the changes, commit the merge with a descriptive message.
# Commit the merge with a message git commit -m "Merge latest changes from main into feature-login"
Step 5: Continue Working on Your Feature:
With the latest changes from the main branch successfully merged into your feature branch, you can continue working on your feature, incorporating the main branch’s updates as needed.
This manual merge process ensures that both branches are up-to-date and conflicts are resolved appropriately, allowing you to maintain code quality and collaboration in your Git workflow.
Creating a Pull Request
Scenario: You’ve completed your work on the “feature-login” branch, and you want to integrate your changes into the main branch using a pull request.
Step 1: Push the Feature Branch:
First, ensure that your feature branch is up-to-date and all your changes are committed. Then, push the feature branch to the remote repository.
# Push the feature-login branch to the remote repository git push origin feature-login
Step 2: Create a Pull Request:
Visit your Git hosting platform (e.g., GitHub, GitLab, Bitbucket) and create a pull request from the “feature-login” branch into the main branch. Provide a meaningful title and description for your pull request, explaining the changes you’ve made.
Step 3: Review and Collaboration:
Your team members and collaborators can now review your pull request. They can leave comments, suggest improvements, and discuss any necessary modifications.
Step 4: Automated Testing:
Many Git hosting platforms offer automated testing and continuous integration (CI) pipelines. These pipelines can run automated tests to ensure that your changes do not introduce errors or regressions.
Step 5: Documentation and Discussion:
Use the pull request as a space for documenting your changes and discussing design decisions. This fosters collaboration and ensures that everyone understands what’s being done.
Step 6: Merge via Pull Request:
Once your pull request has been reviewed, tested, and approved by your team, you can merge it into the main branch using the Git hosting platform’s interface.
By following this workflow, you ensure a controlled and collaborative approach to integrating changes into the main branch. It also allows you to keep your feature branches up-to-date with the latest code from the main branch, promoting code quality, collaboration, and transparency.
Conclusion
Mastering Git is fundamental for efficient and collaborative software development. The concepts of cloning, branching, staging, committing, merging e pull requests are essential for managing projects in an organized, collaborative, and secure manner. They ensure effective version control and facilitate teamwork, ultimately enhancing the development process.